i planing to write about the engine infrastructure and also its API's in the Future.
Cocos2d-x is huge engine so don't expect to know all , but you will learn enough to get started.
1. Change the cocos2d-x-3.8.1\NetBeansProjects\SnakeJS\public_html\index.html file
So the game canvas will be in proportional size and not stretched all over .
index.html:
NetBeansProjects\SnakeJS\public_html\index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>SnakeJS</title> <link rel="icon" type="image/GIF" href="res/favicon.ico"/> <meta name="viewport" content="width=480, initial-scale=1"> <meta name="apple-mobile-web-app-capable" content="yes"/> <meta name="full-screen" content="yes"/> <meta name="screen-orientation" content="portrait"/> <meta name="x5-fullscreen" content="true"/> <meta name="360-fullscreen" content="true"/> <style> body, canvas, div { -moz-user-select: none; -webkit-user-select: none; -ms-user-select: none; -khtml-user-select: none; -webkit-tap-highlight-color: rgba(0, 0, 0, 0); } </style> </head> <body style="text-align: center; padding:0; margin: 0; background: #FFFFFF;"> <script src="res/loading.js"></script> <div style="display:inline-block;width:auto; margin: 0 auto; background: #000; position:relative; border:5px solid black; border-radius: 10px; box-shadow: 0 5px 50px #333"> <canvas id="gameCanvas" width="800" height="450"></canvas> </div> <script src="frameworks/cocos2d-html5/CCBoot.js"></script> <script cocos src="main.js"></script> </body> </html> |
2. Open the file \NetBeansProjects\SnakeJS\public_html\src\app.js
this file will be the game logic starting point .
the file start our main game Scene and Layer , the Layer will hold our game Sprites
they all have Parent child hierarchy relationship to read more about it here:
http://www.cocos2d-x.org/wiki/Scene_Graph
lts clean the file from the starter code the console generated for us :
NetBeansProjects\SnakeJS\public_html\src\app.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | var SnakeJSGameLayer = cc.Layer.extend({ sprite:null, ctor:function () { this._super(); var size = cc.winSize; return true; } }); var SnakeJSScene = cc.Scene.extend({ onEnter:function () { this._super(); var layer = new HelloWorldLayer(); this.addChild(layer); } }); |
3. Do not rush to test your code yet,first we need to change the file: NetBeansProjects\SnakeJS\public_html\main.js
Notice we changed the SnakeJSScene line 13 and SnakeJSGameLayer line 1 variables
We need now to change it also in the main.js file :
NetBeansProjects\SnakeJS\public_html\main.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | cc.game.onStart = function(){ if(!cc.sys.isNative && document.getElementById("cocosLoading")) //If referenced loading.js, please remove it document.body.removeChild(document.getElementById("cocosLoading")); // Pass true to enable retina display, disabled by default to improve performance cc.view.enableRetina(false); // Adjust viewport meta cc.view.adjustViewPort(true); // Setup the resolution policy and design resolution size cc.view.setDesignResolutionSize(800, 450, cc.ResolutionPolicy.SHOW_ALL); // The game will be resized when browser size change cc.view.resizeWithBrowserSize(true); //load resources cc.LoaderScene.preload(g_resources, function () { cc.director.runScene(new SnakeJSScene()); }, this); }; cc.game.run(); |
The change is in line 15 , the main.js is our game initializator/ bootstrapper/ dispatcher class,
It responsible to how the screen /view size will be and which pre game configuration to set and more
None game logic related stuff .
Now go ahead and press the green play button in NetBeans and see what you got.
Should look like this :
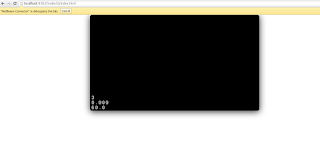
Yep , empty Scene.
4. the game resources are configured in special file called : SnakeJS\public_html\src\resource.js
it contains the image names and paths:
4. the game resources are configured in special file called : SnakeJS\public_html\src\resource.js
it contains the image names and paths:
1 2 3 4 5 6 7 8 9 10 11 | var res = { snakecell_png : "res/snakecell.png", snakefood_png : "res/snakefood.png", blank_png : "res/blank.png" }; var g_resources = []; for (var i in res) { g_resources.push(res[i]); } |
We are ready to start programming our "SnakeJS" game logic in the next tutorial :
Snake Game Using Cocos2d-x HTML5 - PART 3
Play the final SnakeJS game
http://meiry.github.io/SnakeJS-html5/publish/html5/
The SnakeJS source code:
https://github.com/meiry/SnakeJS-html5